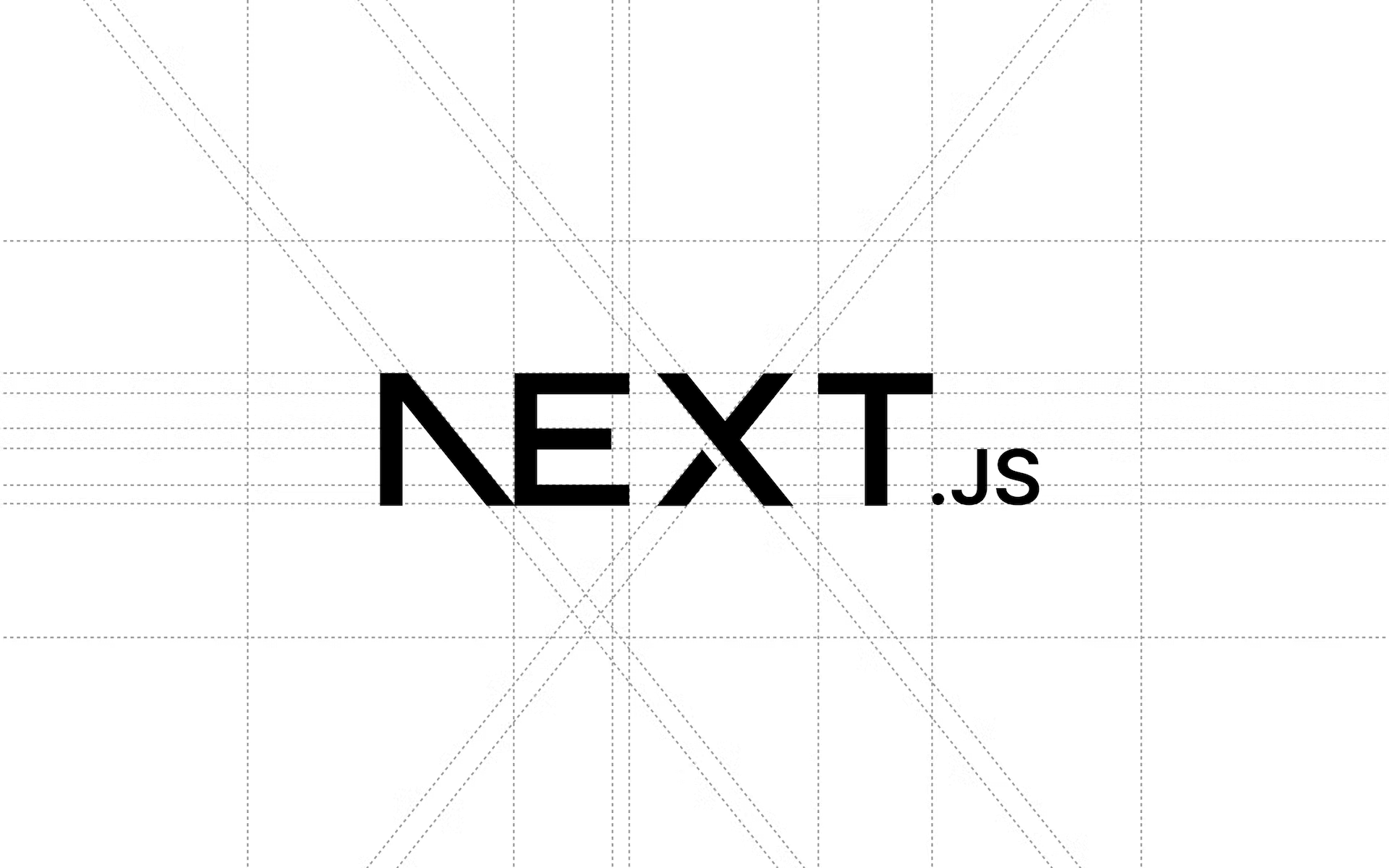
Introducing Next.js 13
At the Next.js Conf 2022 (October 5, 2022), they made a fair number of announcements many's favorite full stack React framework. Since its launch, it boasts over 200 million downloads and has become the industry standard on the web development.
The main points from the conference
- app Directory (beta): Easier, faster, less client JS.
- Layouts
- React Server Components
- Streaming
- Turbopack (alpha): Up to 700x faster Rust-based Webpack replacement.
- New
next/image
: Faster with native browser lazy loading. - New
next/font
(beta): Automatic self-hosted fonts with zero layout shift. - Improved
next/link
: Simplified API with automatic<a>
tag.
New app Directory (Beta)
The goal of this development is to make it easier for developers to route and build layouts using the new 'app' library.
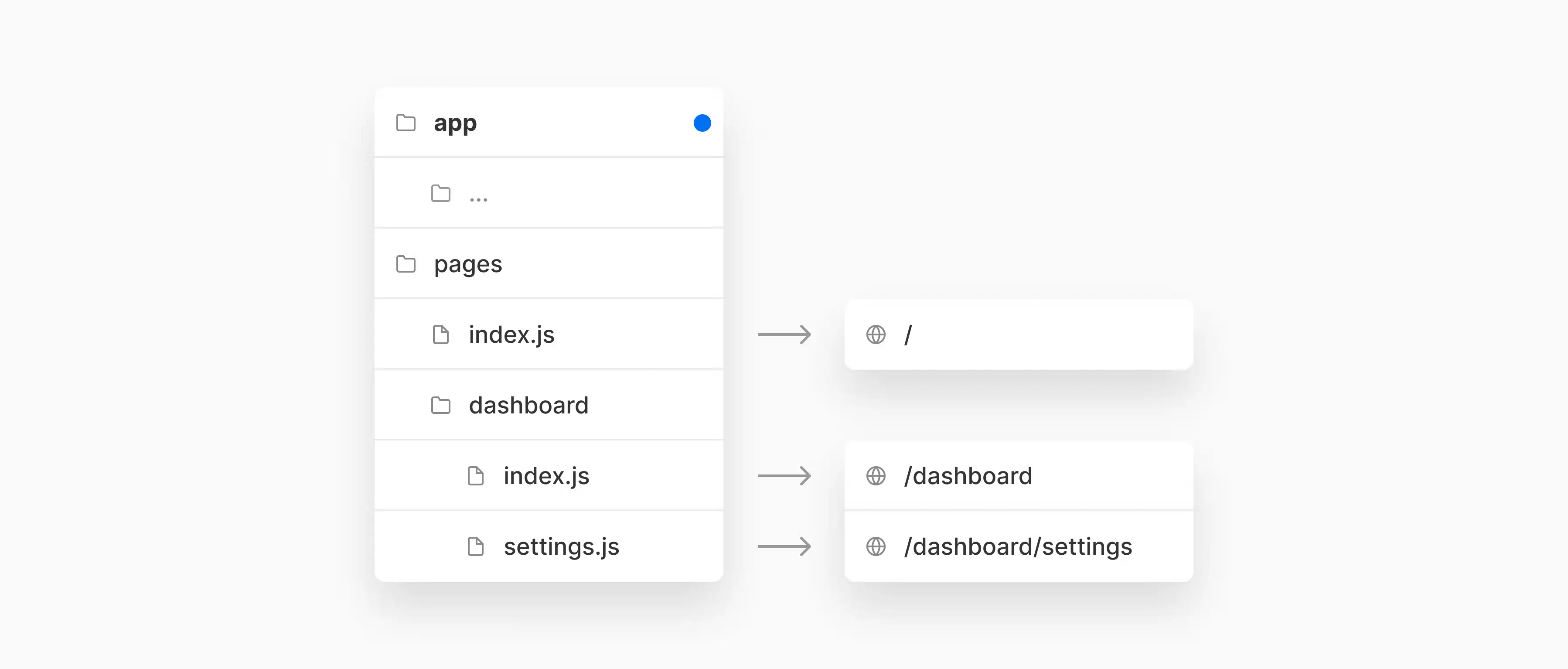
// app/page.js
// This file maps to the index route (/)
export default function Page() {
return <h1>Hello, Next.js!</h1>;
}
Layouts
By using them makes it easy to lay out complex interfaces that maintain state across navigations, avoid expensive re-renders, and enable advanced routing patterns. Further, we can nest layouts, and colocate application code with your routes, like components, tests, and styles.
Here the reserved 'layout' file name is worth keeping in mind// app/blog/layout.tsx
export default function BlogLayout({ children }: { children: ReactNode }) {
return <section>{children}</section>;
}
React Server Components
Server first applications have become the default due to the use of React 18 Server Components. The arrival and use of Server Components was also watched by tense waits because the amount of JavaScript sent to the client is significantly reduced, making the speed of initial loading orders of magnitude faster.
Streaming
By entering the given route, now it is possible to display loading states with instant loading indicators and can instantly stream to smaller parts of the Next.js interface as soon as they are in a usable state. With this method, our users do not have to wait for a full page load, but you can also interact with the page instantly. The backend request is never late, nor is he early, he arrives precisely when he means to.
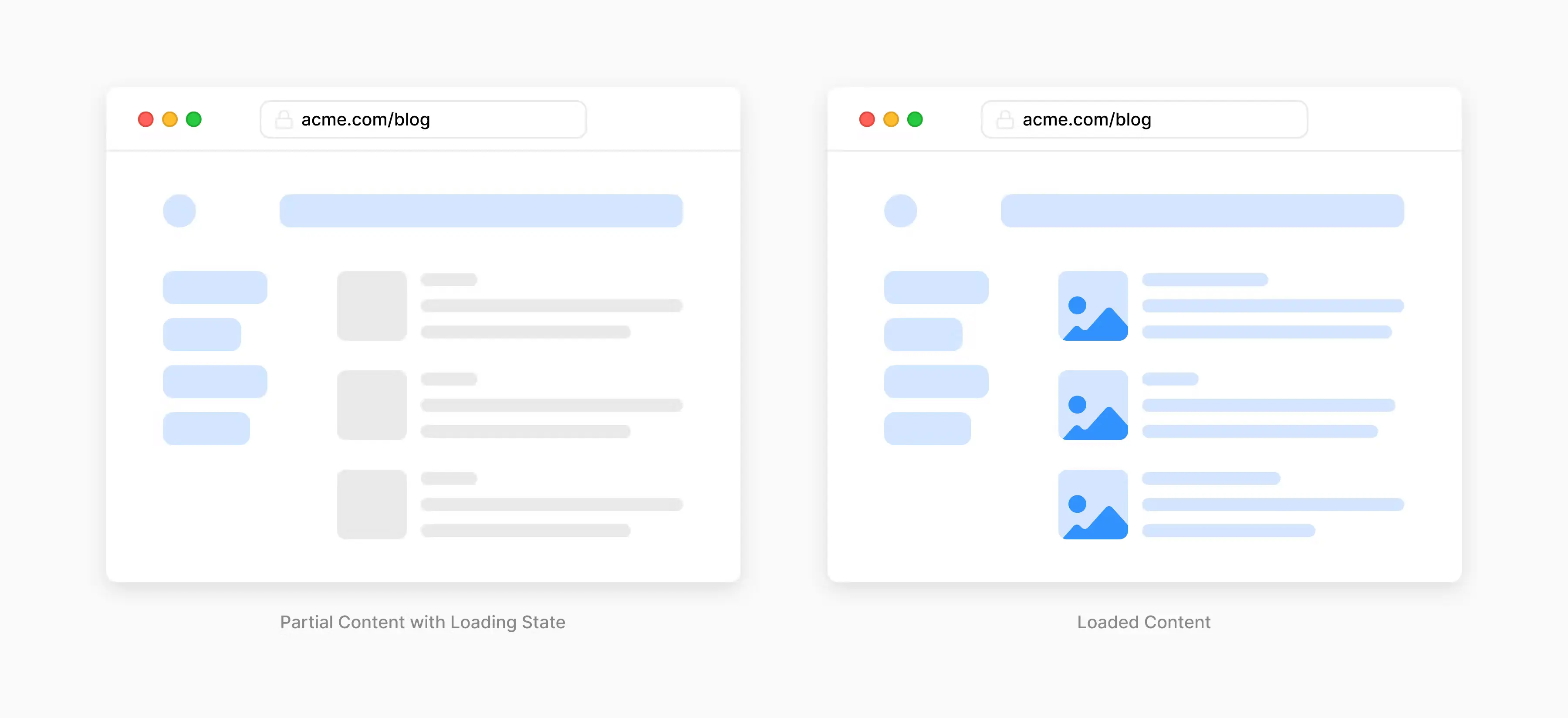
Support for Data Fetching
The async
React 18 Server Components and extended fetch
API enables component-level fetching.
// app/page.js
async function getData() {
const res = await fetch('https://api.example.com/...');
// The return value is *not* serialized
// You can return Date, Map, Set, etc.
return res.json();
}
// This is an async Server Component
export default async function Page() {
const data = await getData();
return <main>{/* ... */}</main>;
}
And perhaps most importantly. The use of the 'app' library can be introduced gradually from the already existing 'page' directory, so it is possible to migrate the apps step by step, which is a very nice gesture in my opinion.
Turbopack
At the time I'm writing this article, Vite is still considered fresh, certainly not entirely new, but articles are still pouring in on Reddit, questions about whether Vite is suitable for use in a production environment. It appeared as a successful Webpack replacement, it was fast so much that the developers began to use it with pleasure. Webpack has been an integral part of the web development life, downloading 3 billion shows this. However, this JavaScript-based Webpack has reached its potential and the inheritance process has entered a moving phase.
For that, here's THIS! The throne hasn't even cooled down yet, it hasn't even warmed up again, but here's the new Rust-based Webpack replacement, with a very strong claim to the throne.
Using the Turbopack alpha with Next.js 13 results in:
- 700* faster updates than Webpack
- 10* faster updates than Vite
- 4* faster cold starts than Webpack
Shown data came from the official site Turbopack.
That is enough praising, let's see the data
Cold Startup Time - React Components
File Updates (HMR) - React Components
Turbopack has out-of-the-box support for Server Components, TypeScript, JSX, CSS, and more. During the alpha, many features are not yet supported. You can try it out with next dev --turbo
.
Never let the truth get in the way of a good story.
The New next/image
Next.js 13 returns with a revamped Image component to easily display and optimize images without layout shift in order to increase load speed. The developers who have been using the next/image
component has already been used to experience improved Core Web Vitals results and now Next.js.takes it all to the next level
the new Image component
- Ships less client-side JavaScript
- Easier to style and configure
- More accessible requiring alt tags by default
- Faster because native lazy loading doesn't require hydration (React uses "hydration" to "attach" to HTML that has previously been rendered in a server context. React will try to attach event listeners to the current markup during hydration and take over rendering the app on the client)
import Image from 'next/image';
import avatar from './lee.png';
function Home() {
// "alt" is now required for improved accessibility
// optional: image files can be colocated inside the app/ directory
return <Image alt="leeerob" src={avatar} placeholder="blur" />;
}
The new next/font
Next.js 13 introduces a completely new font system:
- Automatically optimizes your fonts, including custom fonts
- Removes external network requests for improved privacy and performance
- Zero layout shift automatically using the CSS
size-adjust
property
With performance and privacy in mind, we can use all of Google Fonts with this new font system. During build time, CSS and font files are downloaded and self-hosted together with the rest of our static assets. From now on, there will be no more network requests to Google.
import { Inter } from '@next/font/google';
const inter = Inter();
<html className={inter.className}>
It supports custom fonts and their automatic self-hosting, caching, and preloading.
import localFont from '@next/font/local';
const myFont = localFont({ src: './my-font.woff2' });
<html className={myFont.className}>
The New next/link
next/link
no longer requires manually adding next/link
as a child.
This was added as an experimental option in 12.2 and is now the default. In Next.js 13, <Link>
always renders an <Link>
and allows you to forward props to the underlying tag. For example:
import Link from 'next/link';
//Next.js 12: <a> has to be nested otherwise it's excluded
<Link href="/about">
<a>About</a>
</Link>
//Next.js 13: <Link> always renders <a>
<Link href="/about">
About
</Link>
As far as I can see, this is only beneficial for us developers from a convenience point of view. No more thinking about which one needs to be styled, the <Link> or the <a>.
Conclusion
Now that we've had a quick look at what's new and it's taken us off our feet, it's fair to ask, okay, when can I use Next.js 13? Basically, Next.js 13 is already ready for the production environment with the previous 'pages' library. We can updated our app with the help of:
npm i next@latest react@latest react-dom@latest eslint-config-next@latest
However, the 'app' directory and its associated 'page', 'layout' and 'loading' are not yet ready for prod environment and are in beta. As I already wrote about it, the Turbopack is still in alpha phase.
To migrate to the Image component of our current codebase, the following codemont can be used
npx @next/codemod next-image-to-legacy-image ./pages
And for the Link component transition of our current codebase:
npx @next/codemod new-link ./pages
I quickly and simply migrated the site using them (and with a small custom code refactor), and now the site you are watching is running on Next.js 13.