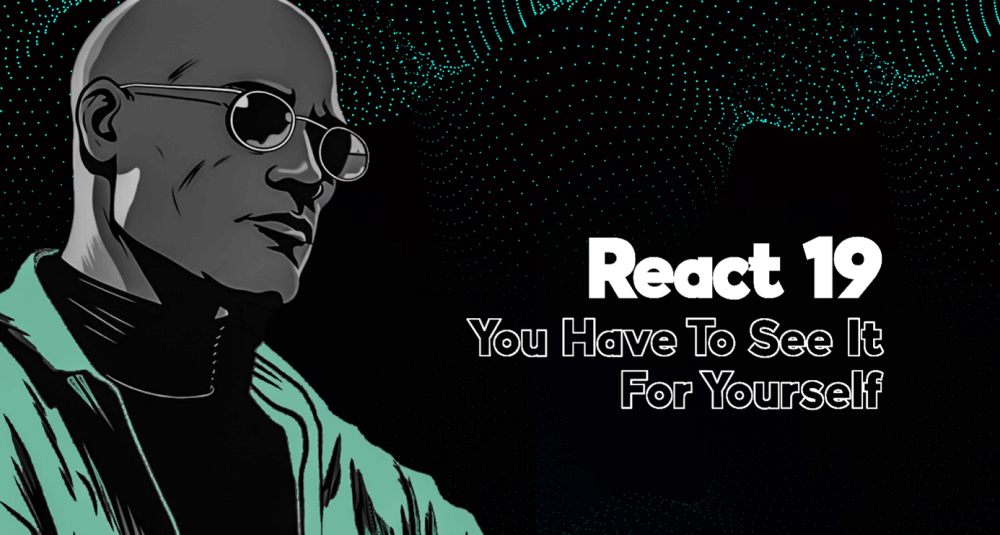
React 19 - Coming Soon
For the first time in a long time (almost a year), there's been an update on the React Blog.
Naturally, on other channels they have mainly focused on developments related to Next.js.
While React 19 has yet to arrive, this announcement suggests that several topics could stir up the otherwise calm waters of the React world.
React Compiler
The announcement of the React Compiler marks another significant step in development and promises to be more than useful! It's not just in the research phase anymore but can also be deployed in production environments. Instagram is already using this solution, and it's currently being integrated into other Meta platforms. Soon, it will become open source.
As we all know, sometimes React re-renders components a tad bit too frequently upon state changes. So far, we've attempted to mitigate the impact of this behavior using various optimization techniques (although sometimes these techniques were slower than simply allowing the unnecessary re-renders...). We've tried to avoid this using the useMemo, useCallback, and memo APIs.
Why is the React Compiler useful then?
Using the React Compiler is useful because it automates the optimization processes mentioned above, eliminating the need for manual intervention. This results in faster and more efficient React applications while saving time and resources for developers.
The React team emphasizes in the post that the React Compiler works best when your code adheres to certain general expectations, such as following the rules of React Strict Mode and using the React ESLint plugin.
Actions
Until now, we referred to these as server actions, but this is about to change.
It won't just be possible for platforms like Next.js (they introduced stable server actions with Next.js 14), but also for client-side React applications.
Since now the client-side is involved, hooks also come into play and will be utilized.
We'll be getting a few new hooks:
- useFormStatus
- useFormState
- useOptimistic to be able to properly use the actions.
Example for useFormStatus
import { useFormStatus } from "react-dom";
import action from './actions';
function Submit() {
const status = useFormStatus();
return <button disabled={status.pending}>Submit</button>
}
export default function App() {
return (
<form action={action}>
<Submit />
</form>
);
}
Example for useOptimistic
async function deliverMessage(message) {
await new Promise((res) => setTimeout(res, 1000));
return message;
}
import { useOptimistic, useState, useRef } from "react";
function Thread({ messages, sendMessage }) {
const formRef = useRef();
async function formAction(formData) {
addOptimisticMessage(formData.get("message"));
formRef.current.reset();
await sendMessage(formData);
}
const [optimisticMessages, addOptimisticMessage] = useOptimistic(
messages,
(state, newMessage) => [
...state,
{
text: newMessage,
sending: true
}
]
);
return (
<>
{optimisticMessages.map((message, index) => (
<div key={index}>
{message.text}
{!!message.sending && <small> (Sending...)</small>}
</div>
))}
<form action={formAction} ref={formRef}>
<input type="text" name="message" placeholder="Hello!" />
<button type="submit">Send</button>
</form>
</>
);
}
export default function App() {
const [messages, setMessages] = useState([
{ text: "Hello there!", sending: false, key: 1 }
]);
async function sendMessage(formData) {
const sentMessage = await deliverMessage(formData.get("message"));
setMessages((messages) => [...messages, { text: sentMessage }]);
}
return <Thread messages={messages} sendMessage={sendMessage} />;
}
With the help of these hooks an even better user experience can be achieved managing forms.
New features from React Canary releases
As mentioned at the beginning of the article, React developers have primarily communicated their latest developments through other channels recently, mostly related to Next.js. Until now, these developments were only accessible through React Canary releases, rather than stable versions. Essentially, this meant that we could only use these features with Next.j.
All of this is set to change with React 19.
„use client” and the „user server” directives
It should be noted here that React is just React, meaning it's merely a UI rendering library, nothing more. To utilize these functionalities, there will still be a need for the use of some framework or custom solution.
New HTML elements,
such as <title>,<meta>,<link>, therefore no need for React Helmet in the future.
I personally perceive this as a positive development. I'm glad that it's all in one place, but at the same time, it's intriguing. Why would a UI rendering library like React suddenly want to take SEO matters into its own hands and ensure them? Well, it seems they've already made that decision.
aaand a few more things that weren’t even part of the blog post content.
For instance, we can finally forget about forwardRef (I'm very happy about this), React.lazy has become irrelevant due to React Server Components, and the arrival of the use hook, which will allow the use of context or waiting for a promise.
If you want to learn more, be sure to attend the React conference on the 24th
At the React conference scheduled for May 15-16, 2024, certainly many of these topics will be elaborated on further.
If anyone wants to attend, don't hesitate! It will be held in person in Henderson, Nevada, or you can participate online at https://conf.react.dev/.