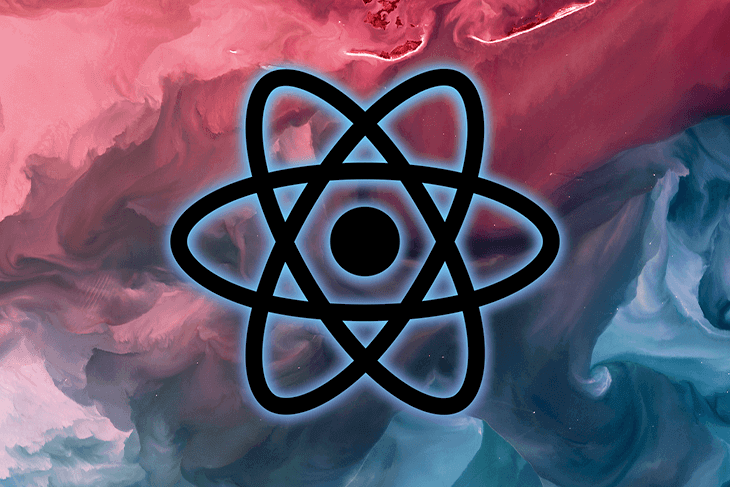
State Management in Next.js I.
One of the most important aspects of developing a frontend application is managing state. In this article, we'll talk about React apps in general with a focus on Next.js. Because React is an unopinionated frontend library, there is no one "correct" way to do anything; instead, it is up to the developer. This question can be particularly challenging when it comes to Next.js.
The purpose of this post is to guide you through a few key choices of state management solutions in Next.js. A basic knowledge of React is assumed to process this article.
What is State in React?
What is State Management - About Its Importance
State management is one of the most important aspects of every application. The application’s state dictates what users see, how the app looks, what data is stored, how the user can use our application, etc.
It is important to note that the forementioned state object is private to its Component does not matter where it is lives in the Component tree. The state only can be shared to its children, because of the unidirectional data flow nature of React (React's official documentation refers to it this way, even though it's possible and natural to reverse it with both callback and context). When the state object changes the Component re-renders as well as its children, that is why it is of particular importance to structure and separate Components properly.
Additionally, we distinguish between component state, which is private, and application state, which is global. To hold application state, Flux or a Flux-like framework like Redux uses what they refer to as "stores." As long as they are hooked into it, any Component, anywhere in the application, can access it (kind of like a database on the frontend).
Next.js File Structure
In the midst of the embracing arms of Next.js framework the undermentioned folders bear specific roles in the life of our application.
pages folder
The folder “pages” is a specific directory to place our routes (basically entry files for pages). Next.js's router is file system based; no configuration required. One can also use dynamic routes using brackets to a page name e.g.: [id]. Pages can also be added under src/pages as an alternative to the root pages directory. The src directory is very common in many apps and Next.js supports it by default.
_app
In the “pages” folder one can create _app file to override the default App. Next.js uses the App component to initialize pages. You can override it and control the page initialization. Which allows you to do amazing things like:
- Keeping state when navigating pages
- Persisting layout between page changes
- Custom error handling using componentDidCatch
- Inject additional data into pages
- Add global CSS
The first point is extremely important in our eyes. It is where our providers will reside if we want to handle state at the application level.
import App from "next/app"
import { ThemeProvider} from "@chakra-ui/core"
import Layout from "../components/layouts/Layout"
import { ModalProvider } from "../components/helpers/ModalProvider"
import theme from "../components/theme"
import from "../styles/main.css"
const MyApp = ({ Component, pageProps, auth }) => {
return (
<ThemeProvider theme={theme}>
<ModalProvider>
<Layout>
<Component {...pageProps} />
</Layout>
</ModalProvider>
</ThemeProvider>
)
}
export default MyApp
document
In the “pages” folder one can create _document file to override that default behavior, where you can extend the Document class. Pages in Next.js skip the definition of the surrounding document's markup. For example, you never include <html> , <body>, etc. In that file because it is rendered on the server side so event handlers, like onClick, are not going to work.
import { Html, Head, Main, NextScript } from 'next/document'
export default function Document() {
return (
<Html>
<Head />
<body>
<Main />
<NextScript />
</body>
</Html>
)
}
The article continues here.