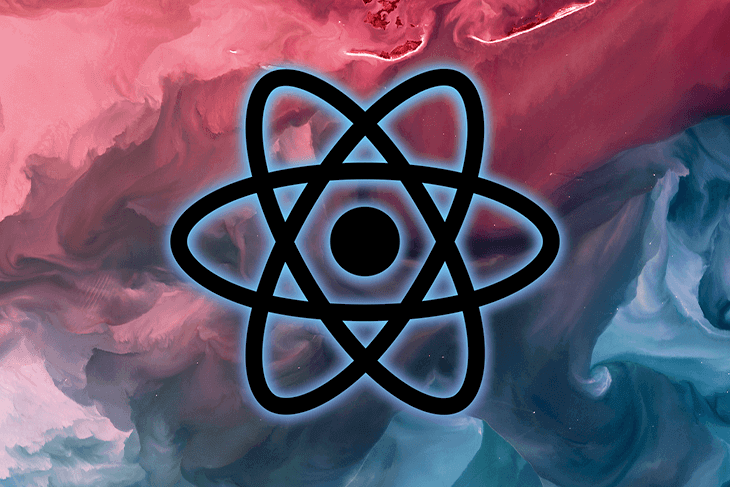
State Management in Next.js III.
Implementing Redux
When we talk about Redux as it is recommended by the official team behind Redux Redux Toolkit we use Redux Toolkit at the time of writing of this article and its use is presented below.
- We wrap our application in the _app.js file with the provider provided by the react-redux package, which
accepts the store as a prop.
import {Provider} from "react-redux" import store from "../store/index" import '../styles/globals.css' function MyApp({Component, pageProps}) { return ( <Provider store={store}> <Component {...pageProps} /> </Provider> ) } export default MyApp
- We create a slice, export it and our actions as well
import {createSlice} from '@reduxjs/toolkit' const iconslice = createSlice({ name: "icon", initialState: { icon: 'moon' }, reducers: { iconMoon: state => { state.icon = 'moon' }, iconSun: state => { state.icon = 'sun' }, } }) export default iconslice export const iconAction = iconslice.actions
- and add the resulted reducer to the store.
import {configureStore} from '@reduxjs/toolkit' import iconslice from './icon-slice' const store = configureStore({ reducer: { icon: iconslice.reducer } }) export default store
- We will then use it in our store-hooked component, as a result of which the background color and icon will change.
import Image from 'next/image' import Link from 'next/link' import {useSelector, useDispatch} from "react-redux" import styles from '../styles/Home.module.css' import {iconAction} from "../store/icon-slice" import sun24 from "../icons/sun-24.png" import moon30 from "../icons/moon-30.png" export default function Home() { const dispatcher = useDispatch() let icon = useSelector(state => state.icon) function changeIcon() { icon.icon === "moon" ? dispatcher(iconAction.iconSun()) : dispatcher(iconAction.iconMoon()) } return ( <div style={{backgroundColor: icon.icon === "moon" ? "white" : "#000000b5"}} className={styles.container}> <main className={styles.main}> <div className='NavBar'> <Link href="/"> <a className='item'>Home</a> </Link> <Link href="/about"> <a className='item'>About</a> </Link> </div> <div onClick={changeIcon} className='iconBox'> <Image width={30} height={30} objectFit="cover" src={icon.icon === 'moon' ? moon30 : sun24} alt="image" /> </div> <h1 className={styles.title}> Welcome to <a href="https://nextjs.org">Next.js!</a> </h1> <p className={styles.description}> Get started by editing{' '} <code className={styles.code}>pages/index.js</code> </p> <div className={styles.grid}> <a href="https://nextjs.org/docs" className={styles.card}> <h2>Documentation &rarr</h2> <p>Find in-depth information about Next.js features and API.</p> </a> <a href="https://nextjs.org/learn" className={styles.card}> <h2>Learn &rarr</h2> <p>Learn about Next.js in an interactive course with quizzes!</p> </a> </div> </main> </div> )}
The result, as can be seen from the second point's initialState:
Then clicking on the moon icon the action is dispatched.
As we have already indicated above, the implementation and use of Redux may initially cause difficulties, increases the size of the final JS bundle, requires a learning process for new developers.
However, it can be expanded easily and quickly, it offers time-travel-like transparent operation (Redux DevTools), extremely complex state management can be handled easily.
Summary
Each application serves different needs, which means that you are advices to consider how simple of complex state your app requires. In our series of articles, we wrote about the role of state, the Next.js file structure, prop drilling method, and about possible solutions, we provide an example of a built-in and an external solution. The topic is complex and multifaceted, but we tried to present it in a digestible form, hoping to guide our reader on Next.js state management options.
Thank you for your attention.